-
Chapter 1: HTML and CSS Interaction
- Website Organization
- Organization and Usability
- HTML Document Structure: The Box Model
- Box Model Example CSS Style
- Color Review
- Interactive Pseudo-Elements
- Non-semantic box elements
- ID and class attributes for finer grained styling application
- CSS .classname a #id_name
- Cell Phone User Interface Priorities
- Chapter 2: Javascript and DOM Basics
-
Chapter 3: Javascript Concepts for React Native
- Javascript History
- React History
- Topics
- Debug Console
- JSON Objects
- Convert between string and JSON object
- Var and React Native
- Javascript 2015 Classes
- Arrays and “destructured” assignment of elements
- In case you’re curious, destructured JSON Objects
- Javascript functions, old and new
- Map: A “Higher Order Function”
- Anonymous (lambda) functions and Map
- Looking Ahead: Use React to Display A List Within an XML Element
- Two option if-else branch
- Nifty List Shortcut: Map and ? : if-else
- Chapter 4: React Native Environment
-
Chapter 5: React Native App Basics
- Topics
- React Native Motivation
- React History
- Creating and Running a React Native App
- Add a JSX element to App.js
- React Native Components
- Composite Design Pattern
- Virtual Document Object Model
- Virtual DOM for Improved Performance
- React Native JSX Components Instead of HTML Elements
- React Babel Compiler Bridges the gap between Javascript and JSX
React Native Programming by Alisa Neeman
Chapter 1:
- Web page folder
- CSS subfolder
- Javascript subfolder
- Images subfolder
- Relative addressing - you may move your website to a different location on the server, or a different server, but link still works
href="css/style.css"
href="images/logo.jpg"
js images css Site.html My Site - Separate document structure and document design (look-and-feel)
- Designs that work on a variety of screen sizes
- Readable font size
- Easily navigable/clickable as aspect ratio (width to height) changes
- Mobile first design
- Add dynamic responses to user interaction
Margin: distance from enclosing element’s edge border
Padding: distance from border
Content area with width and height attributes
p {
margin:50px;
padding:10px;
background-color:AliceBlue;
/* If you forget the semicolon, attribute will be omitted */
border:3pt;
border-color:MediumBlue;
border-style:dotted solid;
/* top & bottom dotted, left & right solid */
text-align: center;
font-family:Helvetica,Arial,sans-serif;
color:Black;
}
- Specify named color background-color:AliceBlue;
- Specify Red, Green and Blue components in hexadecimal digits: #
RRGGBB
background-color:#ff6347;
- CSS style can be applied to an action associated with an HTML element
- Users like to see the effects of their actions: it helps them navigate.
- hover mouse is hovering over element
- active clicked on
- checked when a checkbox is selected
li:hover {
background-color:blue;
font-weight:bold;
}
- When you just want to decorate an arbitrary section of your web page
- "Non-semantic": elements have no meaning such as table or paragraph
- Tag names: < div > and < span >
- Span usually used within a bunch of text content, like a paragraph
- Div creates a line break after the box, span doesn't
- Use id attribute to uniquely identify each element, so each can
have its own unique style (even if the tag types are the same)
<div id="favorite_food">
barbeque
</div>
- Use class attribute when you have multiple elements with the
same style (but maybe not all the elements with that tag type)
<div class="plants">
trees
</div>
<div class="plants">
vines
</div>
- These attributes are often used with div, but can be used with any HTML element
- NOTE: id cannot start with a number, it can start with a letter or underscore, similar to a variable name.
< body >
< div class="important" >
sun
< /div >
< div id="ch1sky" >
blue skies above
< /div >
< div class="important" >
green grass
< /div >
< /body >
.important {
font-size:2em;
}
#sky {
color:white;
background-color:skyblue;
}
- Thumbs are used for scrolling, swiping
- Revert to a single scrollable list of items
- Clickable icons for common tasks placed at bottom
- Be nice to the thumbs!
Chapter 2:
- Firefox: Tools-> Browser Tools-> Web Developer Tools
- Chrome: View-> Developer-> Javascript Console
<script>
var x = “hello”;
x = 5; // variables can change type on the fly
for(i =0; i <5; i++) {
x = x*2;
}
x = [1,2,3];
for ( val in x) {
document.write(x[val]);
// add text to html document
window.alert(x[val]);
// popup
console.log(x[val]);
// f12 activates console
}
</script>
The for val in x loop changes the values to strings. You can also write a traditional Java style for loop in Javascript
x = [1,2,3];
for ( i = 0; i < x.length; i++) {
window.alert(x[i]);
}
<script>
alert(“Hello!”);
if (confirm(“Yes or no?”) ) {
var n = prompt(“Enter text”);
alert(n);
}
</script>
var x = myFunction(4, 3);
function myFunction(a, b) {
return a * b;
}
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.pop(); // Remove the last element ("Mango")
fruits.push("Kiwi"); //Add new last element
fruits[fruits.length] = "Kiwi"; // Appends "Kiwi”
fruits.sort(); // Sorts the elements
fruits.reverse(); // Reverses order of elements
var citrus = fruits.slice(3,5); //(start,stop)
var morefruit = fruits.concat(citrus);//join 2 arrays
var str = "How are you doing today?";
var res = str.split(" "); // split string into
// array of substrings
onchange
An HTML element has been changedonclick
The user clicks an HTML elementonmouseover
The user moves the mouse over an HTML elementonmouseout
The user moves the mouse away from an HTML elementonkeydown
The user pushes a keyboard keyonload
The browser has finished loading the page
var car = {
type:"MINI",
model:"Cooper",
color:"white",
showCar: function() {
alert(this.type + " " +
this.model );
}
};
<!DOCTYPE html>
<html> <head> </head>
<body>
<button onclick="myFunction();"> Click Me</button>
<p id="demo"> </p>
<script>
function myFunction() {
document.getElementById("demo").innerHTML = "Hello World";
}
</script>
</body>
</html>
- Elements are CSS objects
- Methods:
- document.getElementById(id)
- document.getElementsByTagName(name)
- document.getElementsByClassName(name)
- Class variables (properties)
- element.innerHTML = new html content
- element.attribute = new value
- element.style.property = new style
<html>
<head>
<script>
function showHide( y ) {
var x = document.getElementById( y );
//Any HTML element can call a function
// id attribute makes it easy to find the element
// in the document
if ( x.style.display === "none” ) {
x.style.display = "block";
} else {
x.style.display = "none";
}
}
</script>
</head>
<body>
<div id="t1"> Raiders of the Lost Ark</div> <u onclick="showHide('t2')">
More</u>
<div id="t2"> When Dr. Indiana Jones – the tweed-suited professor who just
happens to be a celebrated archaeologist – is hired by the government to locate the
legendary Ark of the Covenant he finds himself up against the entire Nazi regime.
</div>
</body>
</html>
<!DOCTYPE html>
<html>
<head> </head>
<body>
<button onclick="myFunction();" name="mybutton"> Click Me</button>
<p id="demo"> </p>
<script>
function myFunction() { // get first button in page
document.getElementsByTagName("button")[0].innerHTML = "Hello World";
}
</script>
</body>
</html>
Chapter 3:
- September 1995: Netscape (now Firefox) introduced Javascript, competing with Java in the browser window
- 2005:
- Ajax enables files to be loaded in the background (asynchronously)
- Appearance of many helper libraries such as jQuery, MooTools, Dojo
- 2008: Chrome browser with Javascript JIT compiler to improve performance
- 2009: Server side node.js
- 2015: ECMA script 6. class, import, export, arrow functions
- 2016: await async to replace AJAX
- 2009: Chrome’s Node.js released: open source Javascript web server
- 2011: Facebook’s React created, used for newsfeed and Instagram
- 2013: React goes open source. One of many Javascript libraries such as JQuery, etc.
- Object oriented
- Uses JSX compiler extension to convert objects to HTML elements
- Uses Babel compiler to enable Javascript interpretation without <script> tags
- 2015: React Native released. A library for cross platform Mobile and VR apps. JSX provides native XML elements, similar to HTML.
- 2019: React Hooks released A way to share state across non-OOP UI components. (Library moves from OOP to functional programming model)
- JSON objects
- Var and const
- Javascript classes
- Destructured assignment
- Arrow functions
- Map
- Anonymous functions
- If-else using ?:
- Firefox: Tools->Browser Tools->Web Developer Tools
- Chrome: View->Developer->Javascript Console
- JSON stands for JavaScript Object Notation
- Set of name:value pairs
var joeData = { "name":"Joe", "age":20, "car":null };
console.log(joeData.name);
// Use dot notation to get
const obj =
JSON.parse(‘{"name":"Joe", "age":20, "city":"New York"}’);
console.log(obj.age);
var myString = JSON.stringify(obj);
alert("object string " + myString);
What is the difference between console.log and alert?
Component Exception
Can't find picArray
Meaning: you need to use var
to establish the scope block for the
variable declaration.
Then check whether the variable is used in the block or a sub-block of
the declaration.
class Car {
constructor(brand) {
this.carname = brand;
}
present() {
return "I have a " + this.carname;
}
}
var car = new Car("tesla");
console.log(car.present());
The destructuring assignment syntax makes it possible to unpack values from arrays, or properties from objects, into distinct variables. Reduces the number of assignment statements.
[a, b] = [3, 4];
console.log(a);
console.log(b);
const [firstElement, secondElement] = list;
const objA = {
prop1: 'foo',
prop2: {
prop2a: 'bar',
prop2b: 'baz',
},
;
// Deconstruct nested props. Field names
// and curly braces must match object.
const {
prop1,
prop2: {
prop2a, prop2b
}
} = objA;
console.log(prop1); // 'foo'
console.log(prop2a); // 'bar'
console.log(prop2b); // 'baz'
function add (x, y) {
return x + y;
}
New “Arrow” function: less typing
name = (parameters) =>
{ expression(s) ; return value;}
const add = (x, y) => x + y;
return
optional if just a single expression. Use empty parentheses () if no parameters.
In both types, a return value is not required.
Function call is the same, either way.
console.log( add(3,4) )
map
returns new list. (Original list is unchanged).
const numbers = [4, 9, 16, 25];
const newArr = numbers.map(Math.sqrt)
console.log(newArr);
Parameter to map is unnamed arrow function.
(parameter(s)) => expression
Goal: less typing, easier to read.
Your function needs to have a return value for map.
Again, function is passed in as a parameter.
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map( (number) => number * 2 );
console.log(doubled);
const numbers = [1, 2, 3, 4, 5];
const listItems =
numbers.map((num) => {num} );
x = 5;
if (x == 4) {
y = "four";
}
else {
y = "not four";
}
console.log(y);
x = 5;
y = x == 4? "four" : "not four";
console.log(y);
var mylist = [ 1, 0, 1, 1, 0 ];
var L2 = mylist.map(
(num) => num == 1? "A 1!" : "Nada");
console.log(L2);
Chapter 4:
Windows | Linux | Mac | Purpose |
---|---|---|---|
Node.js | Node.js | Node.js | Web server |
Android Studio | Android Studio | Xcode tools | Phone emulator |
Watchman | Watchman | Display updater | |
Expo | Expo | Expo | Ties server and emulator together to create an IDE |
Yarn | Yarn | Yarn | Update packages |
- Say yes to kotlin update
- Create a basic project, with default settings.
- Click the cube icon, click Android 11(API 30) and Android 10 (API 29), in addition to Android 12. Wait for downloads to complete.
- Click the phone icon (your virtual devices)
- + create virtual device
- I would pick a lower resolution device to match the screen of your computer, like nexus 4. Download both 30 and 29. Wait for downloads to complete.
- Click the folder with three blue square icon->Modules. select 29 for Compile Sdk version and 30 build tools version. Wait for downloads.
- Click the green triangle and your phone should open and say “Hello first fragment”.
Say yes to install build tools and chocolatey.
Android Studio
open and run updates
Make a project
Run->Select Device ->stuff to download and install
Create a project and set to version 29 instead of 31.
Expo:
npm install --global expo-cliExpo Install docs
React Native Environment General Info
Getting Started With React Native
Yarn:
npm install --global yarn
- Node.js
-
- Linux:
sudo apt-get install npm
sudo npm install –g n
sudo n latest
-
Mac alternative:
brew update
brew install node
- Linux:
- Watchman
Linux:sudo apt-get install watchman
Mac:brew install watchman
- Expo:
sudo npm install -g react-native-cli
sudo npm install --global expo-cli
Getting started with Expo - Yarn:
npm install --global yarn
- General Info on environment setup
- If using android studio, start a phone emulator first.
- On Mac,
open –a Simulator.app
- Use a command shell with node and expo installed.
- Use expo to create an app starter folder
expo init project-name cd project-name expo start
- Choose Local connection
- Choose a simulator or web browser for the app to run in
- Atom Code Editor
- Click Download
Chapter 5:
- React Native history
- Creating and running a basic app
- JSX, model and design pattern
- Embedded Javascript expressions
- One set of code, works for multiple mobile platforms (iPhone, Android)
- Leverage native performance of mobile platform: speed achieved from running compiled code directly on hardware, instead of through an interpreter.
- Use existing language(s). Includes Javascript + CSS + HTML-like syntax for the user interface.
- 2009: Chrome’s Node.js released: open source Javascript web server
- 2011: Facebook’s React created, used for newsfeed and Instagram
- 2013: React goes open source. One of many Javascript libraries such as JQuery, Dojo, etc.
- Object oriented
- Uses JSX compiler extension to convert objects to HTML elements
- Uses Babel compiler to enable Javascript interpretation without <script> tags
- 2015: React Native released. A library for cross platform Mobile and VR apps. JSX provides native XML elements, similar to HTML.
- 2019: React Hooks released A way to share state across non-OOP UI components. (Library moves from OOP to functional programming model)
- If using android studio, start a phone emulator first.
- Use a command shell with node and expo installed.
- Use expo to create an app starter folder expo init project-name
- Tab down to minimal and hit enter cd project-name expo start
- Choose Local connection
- Choose a simulator or web browser for the app to run in
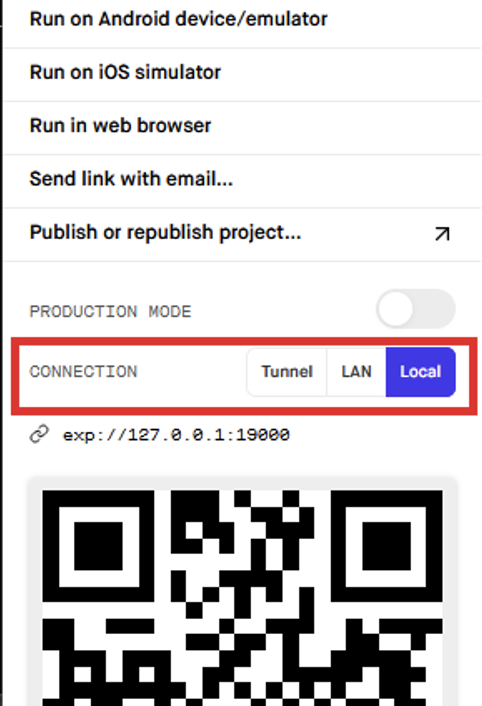
import 'react-native-gesture-handler'; import { registerRootComponent } from 'expo'; import App from './App'; // registerRootComponent calls AppRegistry.registerComponent('main', () => App); // It also ensures that whether you load the app // in Expo Go or in a native build, // the environment is set up appropriately registerRootComponent(App);
export default function App() { return ( <View style={styles.container}> <Text>Open up App.js to start working on your app!</Text> <Text> Hello World </Text> <StatusBar style="auto" /> </View> ); }
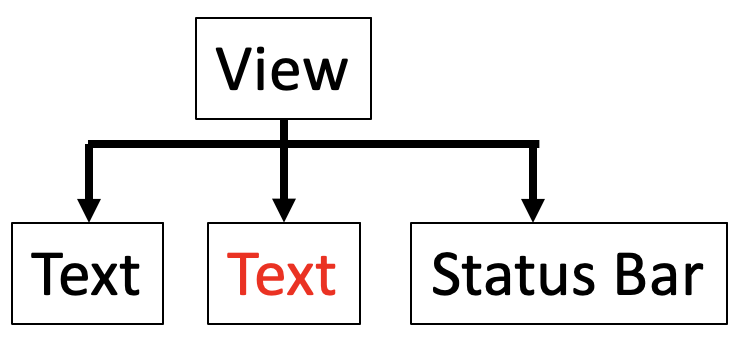
- App.js is a component: a function or React component subclass that returns a JSX element
- In React, it is an HTML tree containing HTML elements and/or React components.
- In React Native it is a JSX tree containing React components.
- An Object can be composed of other objects
- Class variables which are objects
- Suppose those variables also contain objects
- Similarly, A component can be composed of other components
- Composite design pattern
- Compose objects into a tree structure
- Treat individual objects and compositions of objects uniformly
- This means objects and compositions implement the same interface
React Model: One direction of data flow
Data flows in one direction: from parent component to child component.
Component refers to React Component or HTML element.
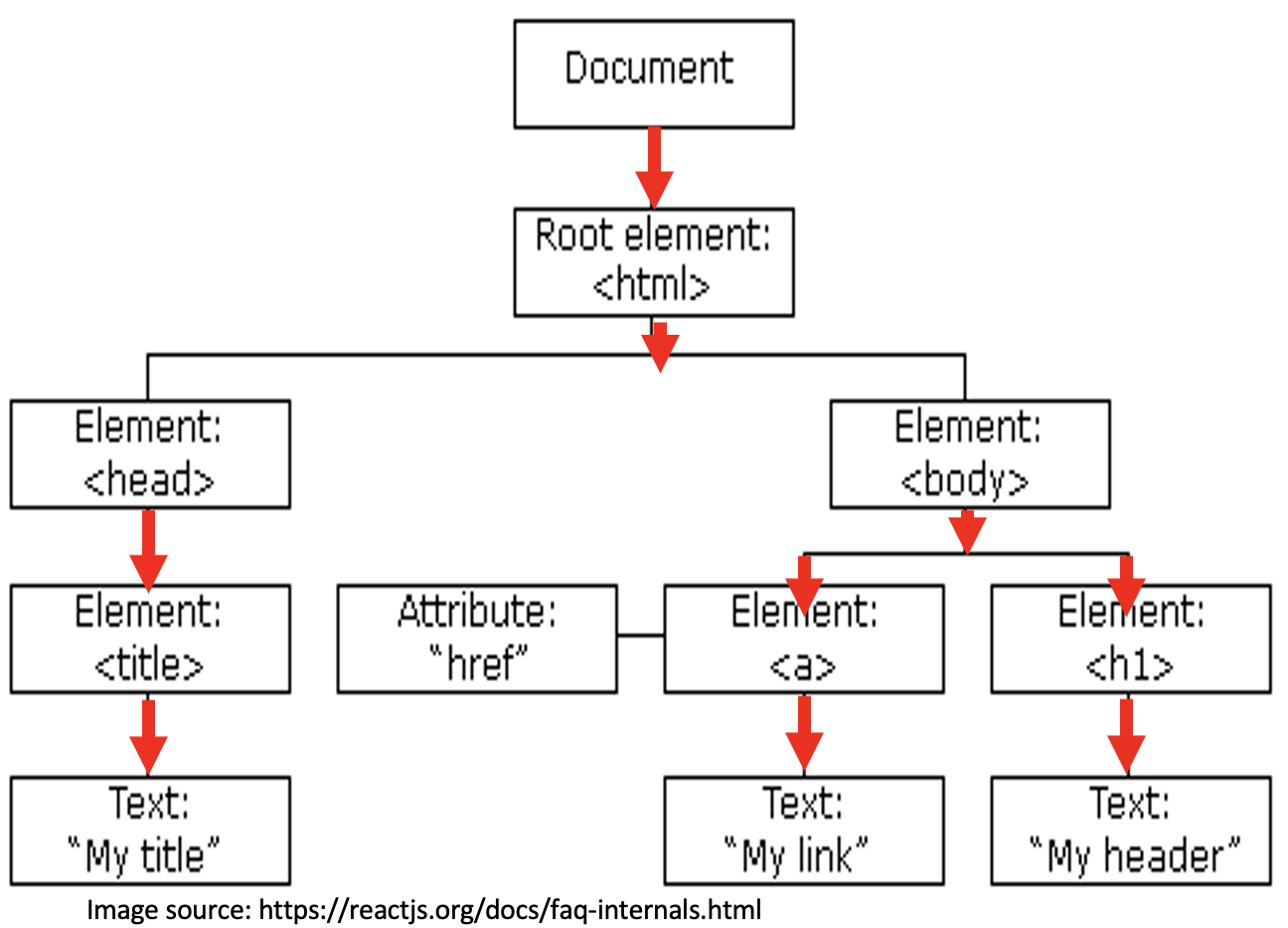

- The virtual DOM is a programming concept where an ideal, or “virtual”, representation of a UI is kept in memory and synced with the “real” DOM, by the ReactDOM library.
- This enables the declarative API of React: You tell React what state you want the UI to be in, and it makes sure the DOM matches that state.
- This abstracts out the attribute manipulation, event handling, and manual DOM updating that you would otherwise have to use to build your app.
- The virtual DOM is a representation of the UI in memory. It is synchronized with the “real” Document Object Model.
- Updates occur quickly, since only necessary subtree changes occur, instead of updating the entire tree.


<Text> {5 + 6} </Text>Result: Less callbacks